Form Contact Send Mail using PHP and Design CSS Bootstrap
1.Index.php
2.Styles.css<?php $result = ""; $error = ""; if(isset($_POST['submit'])) { require 'phpmailer/PHPMailerAutoload.php'; $mail = new PHPMailer; //smtp settings $mail->isSMTP(); // send as HTML $mail->Host = "smtp.gmail.com"; // SMTP servers $mail->SMTPAuth = true; // turn on SMTP authentication $mail->Username = "your email"; // Your mail $mail->Password = 'your password'; // Your password mail $mail->Port = 587; //specify SMTP Port $mail->SMTPSecure = 'tls'; $mail->setFrom($_POST['email'],$_POST['name']); $mail->addAddress('your email'); $mail->addReplyTo($_POST['email'],$_POST['name']); $mail->isHTML(true); $mail->Subject='Form Submission:' .$_POST['subject']; $mail->Body='<h3>Name :'.$_POST['name'].'<br> Email: '.$_POST['email'].'<br>Message: '.$_POST['message'].'</h3>'; if(!$mail->send()) { $error = "Something went worng. Please try again."; } else { $result="Thanks\t" .$_POST['name']. " for contacting us."; } } ?> <!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1"> <title>Contact Form</title> <link href="https://fonts.googleapis.com/css?family=Roboto|Courgette|Pacifico:400,700" rel="stylesheet"> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/font-awesome/4.7.0/css/font-awesome.min.css"> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css"> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.12.4/jquery.min.js"></script> <script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js"></script> <link rel="stylesheet" href="styles.css"> </head> <body> <div class="container"> <div class="row"> <div class="col-md-8 col-md-offset-2 m-auto"> <div class="contact-form"> <h1>Contact Form SendMail</h1> <h2 class="text-center text-white"> <?=$result; ?></h2> <h2 class="text-center text-danger"> <?=$error; ?></h2> <form action="" method="post"> <div class="row"> <div class="col-sm-6"> <div class="form-group"> <label for="inputName">Name</label> <input type="text" class="form-control" id="Name" name="name" placeholder="Enter Full" required> </div> </div> <div class="col-sm-6"> <div class="form-group"> <label for="inputEmail">Email</label> <input type="email" class="form-control" id="Email" name="email" placeholder="Enter Email" required> </div> </div> </div> <div class="form-group"> <label for="inputSubject">Subject</label> <input type="text" class="form-control" id="Subject" name="subject" placeholder="Enter Subject" required> </div> <div class="form-group"> <label for="inputMessage">Message</label> <textarea class="form-control" id="Message" name="message" rows="5" placeholder="Enter Message..." required></textarea> </div> <div class="text-center"> <button type="submit" name="submit" class="btn btn-primary"><i class="fa fa-paper-plane"></i> Send</button> </div> </form> </div> </div> </div> </div> </body> </html>
body { color: #000; font-family: "Roboto", sans-serif; background-image: url("image/background.jpg"); background-repeat: no-repeat, repeat; background-color: rgba(32, 31, 31, 0.212); } .contact-form { padding: 50px; margin: 30px auto; } .contact-form h1 { font-size: 42px; font-family: 'Pacifico', sans-serif; margin: 0 0 50px; text-align: center; } .contact-form .form-group { margin-bottom: 20px; } .contact-form .form-control, .contact-form .btn { min-height: 38px; border-radius: 2px; } .contact-form .form-control { border-color: #e2c705; } .contact-form .form-control:focus { border-color: #d8b012; box-shadow: 0 0 8px #dcae10; } .contact-form .btn-primary { min-width: 250px; color: #fcda2e; background: #000; margin-top: 20px; border: none; } .contact-form .btn-primary:hover { color: #fff; } .contact-form .btn-primary i { margin-right: 5px; } .contact-form label { opacity: 0.9; } .contact-form textarea { resize: vertical; } .bs-example { margin: 20px; }
How to Send Email using PHP mail() Function
What is PHP mail?
PHP mail is the built-in PHP function that is used to send emails from PHP scripts.
The mail function accepts the following parameters;
- Email address
- Subject
- Message
- CC or BC email addresses
- It’s a cost-effective way of notifying users on important events.
- Let users contact you via email by providing a contact us form on the website that emails the provided content.
- Developers can use it to receive system errors by email
- You can use it to email your newsletter subscribers.
- You can use it to send password reset links to users who forget their passwords
- You can use it to email activation/confirmation links. This is useful when registering users and verifying their email addresses
In this tutorial, you will learn-
- Why/When to use the PHP mail
- Simple Mail Transmission Protocol
- Sanitizing email user inputs
- Secure Mail
Why/When to use the mail PHP
Sending mail using PHP
The PHP mail function has the following basic syntax
<?php
mail($to_email_address,$subject,$message,[$headers],[$parameters]);
?>
HERE,
- “$to_email_address” is the email address of the mail recipient
- “$subject” is the email subject
- “$message” is the message to be sent.
- “[$headers]” is optional, it can be used to include information such as CC, BCC
- CC is the acronym for carbon copy. It’s used when you want to send a copy to an interested person i.e. a complaint email sent to a company can also be sent as CC to the complaints board.
- BCC is the acronym for blind carbon copy. It is similar to CC. The email addresses included in the BCC section will not be shown to the other recipients.
Simple Mail Transmission Protocol (SMTP)
PHP mailer uses Simple Mail Transmission Protocol (SMTP) to send mail.
On a hosted server, the SMTP settings would have already been set.
The SMTP mail settings can be configured from “php.ini” file in the PHP installation folder.
Configuring SMTP settings on your localhost Assuming you are using xampp on windows, locate the “php.ini” in the directory “C:\xampp\php”.
- Open it using notepad or any text editor. We will use a notepad in this example. Click on the edit menu
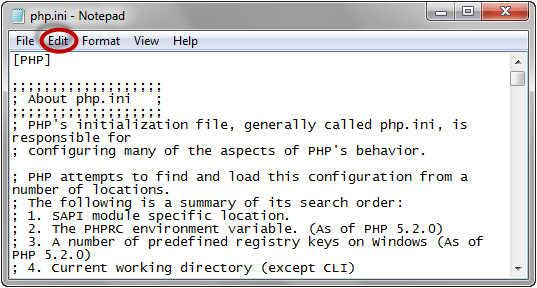
- Click on Find… menu
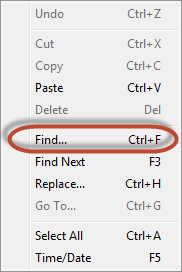
- The find dialog menu will appear
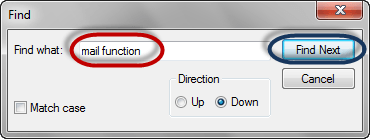
- Click on Find Next button
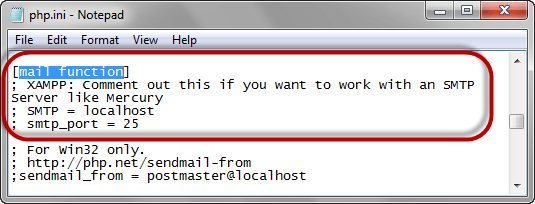
- Locate the entries
- [mail function]
- ; XAMPP: Don’t remove the semi column if you want to work with an SMTP Server like Mercury
- ; SMTP = localhost
- ; smtp_port = 25
- Remove the semi colons before SMTP and smtp_port and set the SMTP to your smtp server and the port to your smtp port. Your settings should look as follows
- SMTP = smtp.example.com
- smtp_port = 25
- Note the SMTP settings can be gotten from your web hosting providers.
- If the server requires authentication, then add the following lines.
- auth_username = example_username@example.com
- auth_password = example_password
- Save the new changes.
- Restart Apache server.
Let’s now look at an example that sends a simple mail.
<?php $to_email = 'name @ company . com'; $subject = 'Testing PHP Mail'; $message = 'This mail is sent using the PHP mail function'; $headers = 'From: noreply @ company . com'; mail($to_email,$subject,$message,$headers); ?>
Output:
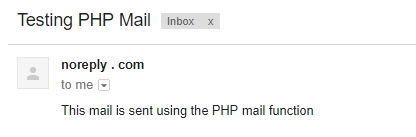
Note: the above example only takes the 4 mandatory parameters.
You should replace the above fictitious email address with a real email address.
Sanitizing email user inputs
The above example uses hardcoded values in the source code for the email address and other details for simplicity.
Let’s assume you have to create a contact us form for users to fill in the details and then submit.
- Users can accidentally or intentional inject code in the headers which can result in sending spam mail
- To protect your system from such attacks, you can create a custom function that sanitizes and validates the values before the mail is sent.
Let’s create a custom function that validates and sanitizes the email address using the filter_var built-in function.
Filter_var function The filter_var function is used to sanitize and validate the user input data.
It has the following basic syntax.
<?php filter_var($field, SANITIZATION TYPE); ?>
HERE,
“filter_var(…)” is the validation and sanitization function
- “$field” is the value of the field to be filtered.
- “SANITIZATION TYPE” is the type of sanitization to be performed on the field such as;
- FILTER_VALIDATE_EMAIL – it returns true for valid email addresses and false for invalid email addresses.
- FILTER_SANITIZE_EMAIL – it removes illegal characters from email addresses. info\@domain.(com) returns info@domain.com.
- FILTER_SANITIZE_URL – it removes illegal characters from URLs. http://www.example@.comé returns >http://www.example@.com
- FILTER_SANITIZE_STRING - it removes tags from string values. <b>am bold</b> becomes am bold.
The code below implements uses a custom function to send secure mail.
<?php
function sanitize_my_email($field) {
$field = filter_var($field, FILTER_SANITIZE_EMAIL);
if (filter_var($field, FILTER_VALIDATE_EMAIL)) {
return true;
} else {
return false;
}
}
$to_email = 'name @ company . com';
$subject = 'Testing PHP Mail';
$message = 'This mail is sent using the PHP mail ';
$headers = 'From: noreply @ company. com';
//check if the email address is invalid $secure_check
$secure_check = sanitize_my_email($to_email);
if ($secure_check == false) {
echo "Invalid input";
} else { //send email
mail($to_email, $subject, $message, $headers);
echo "This email is sent using PHP Mail";
}
?>
Output:
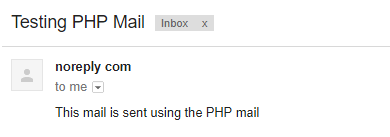
Secure Mail
Emails can be intercepted during transmission by unintended recipients.
This can exposure the contents of the email to unintended recipients.
Secure mail solves this problem by transmitting emails via Hypertext Transfer Protocol Secure (HTTPS).
HTTPS encrypts messages before sending them.
Summary
- The PHP built in function mail() is used to send mail from PHP scripts
- Validation and sanitization checks on the data are essential to sending secure mail
- The PHP built in function filter_var() provides an easy to use and efficient way of performing data sanitization and validation
0 Comments
CAN FEEDBACK
Emoji